【JavaScript】entries()を使って配列のキー/値のペアを取得する方法
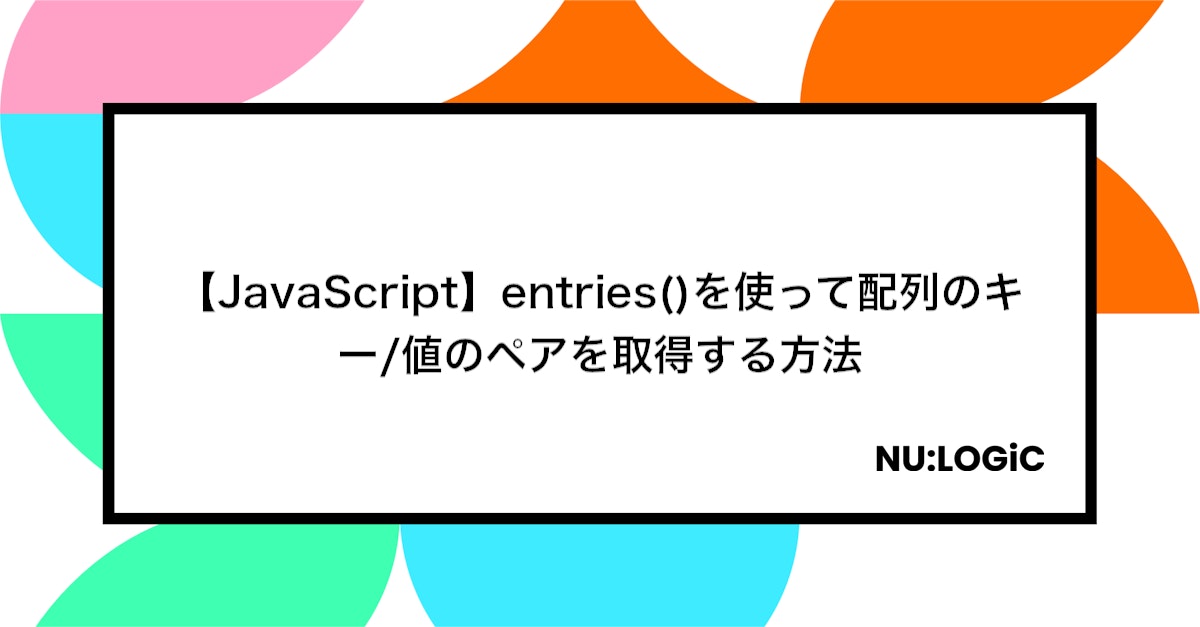
JavaScriptで配列を操作する際に、そのキーと値のペアを取得したいことは多々あります。その際に便利なのがentries()
メソッドです。この記事でentries()
の使い方をわかりやすく解説します。
3行で要約すると
entries()
は配列のキーと値のペアを取得するメソッド- イテレータを返し、for...ofループで使用可能
Object.entries()
はオブジェクトにも似た使い方で適用できる
「entries()」の基本的な使い方
「entries()」とは?
entries()
メソッドは、配列の各インデックスに対するキーとその値のペアを持つ新しいイテレータを返します。これにより、for...ofループでキーと値を同時に簡単に取得できます。
const fruits = ['apple', 'banana', 'cherry'];
for (const [index, fruit] of fruits.entries()) {
console.log(index, fruit);
}
// 0 "apple"
// 1 "banana"
// 2 "cherry"
「entries()」の使用例4パターン
インデックスと要素の取得
キー(インデックス)とその要素を同時に取得したい時に使用します。
const colors = ['red', 'green', 'blue'];
for (const [index, color] of colors.entries()) {
console.log(`Index: ${index}, Color: ${color}`);
}
// Index: 0, Color: red
// Index: 1, Color: green
// Index: 2, Color: blue
配列をオブジェクトに変換
entries()
を使って配列をオブジェクトの形式に変換することができます。
const animals = ['cat', 'dog', 'bird'];
const obj = Object.fromEntries(animals.entries());
console.log(obj);
// {0: "cat", 1: "dog", 2: "bird"}
配列の特定の要素探索
キーと値を使って特定の要素を探すことができます。
const numbers = [10, 20, 30, 40];
for (const [index, num] of numbers.entries()) {
if (num === 30) {
console.log(`Found 30 at index: ${index}`);
}
}
// Found 30 at index: 2
二次元配列の操作
二次元配列での操作も可能です。
const matrix = [[1, 2], [3, 4], [5, 6]];
for (const [rowIndex, [a, b]] of matrix.entries()) {
console.log(`Row: ${rowIndex}, Values: ${a}, ${b}`);
}
// Row: 0, Values: 1, 2
// Row: 1, Values: 3, 4
// Row: 2, Values: 5, 6
「entries()」と「Object.entries()」
「Object.entries()」とは?
オブジェクトのキーと値のペアを配列として取得する際に使用します。
const person = {name: "John", age: 30};
const entries = Object.entries(person);
console.log(entries);
// [["name", "John"], ["age", 30]]
「entries()」と「Object.entries()」の違い
主な違いは、entries()
は配列専用で、Object.entries()
はオブジェクト専用です。
const arr = ['a', 'b', 'c'];
console.log([...arr.entries()]);
// [[0, "a"], [1, "b"], [2, "c"]]
const obj = {0: "a", 1: "b", 2: "c"};
console.log(Object.entries(obj));
// [["0", "a"], ["1", "b"], ["2", "c"]]
「entries()」を使用する際の注意点
ネストした配列
entries()
はネストした配列に対して、1階層のキーと値のペアのみを取得します。深くネストした情報はそのままとなるので注意が必要です。
const nested = [[1, 2], [3, [4, 5]]];
for (const [index, value] of nested.entries()) {
console.log(index, value);
}
// 0 [1, 2]
// 1 [3, [4, 5]]
「entries()」の使い方まとめ
entries()
は配列のキーと値のペアを効果的に操作するための強力なメソッドです。適切な場面で活用することで、より読みやすく効率的なコードを書くことができます。